here the relevant code to the icon numbering
import panel as pn
h = 70
svg_vsc = f""" <svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 48 48" width="{h}px" height="{h}px"><path fill="#29b6f6" d="M44,11.11v25.78c0,1.27-0.79,2.4-1.98,2.82l-8.82,4.14L34,33V15L33.2,4.15l8.82,4.14 C43.21,8.71,44,9.84,44,11.11z"/><path fill="#0277bd" d="M9,33.896L34,15V5.353c0-1.198-1.482-1.758-2.275-0.86L4.658,29.239 c-0.9,0.83-0.849,2.267,0.107,3.032c0,0,1.324,1.232,1.803,1.574C7.304,34.37,8.271,34.43,9,33.896z"/><path fill="#0288d1" d="M9,14.104L34,33v9.647c0,1.198-1.482,1.758-2.275,0.86L4.658,18.761 c-0.9-0.83-0.849-2.267,0.107-3.032c0,0,1.324-1.232,1.803-1.574C7.304,13.63,8.271,13.57,9,14.104z"/></svg>"""
icon_class = 'svg_vsc'
svg = pn.pane.SVG(svg_vsc, css_classes=[icon_class])
html = pn.pane.HTML('')
slider = pn.widgets.IntSlider(name='Counter',value=0,end=100)
html.object = f"""
<script>
console.log(document.getElementsByClassName('{icon_class}')[0]);
let NumberSpan{icon_class} = document.createElement('span');
NumberSpan{icon_class}.style.position = 'relative';
NumberSpan{icon_class}.innerHTML = '';
NumberSpan{icon_class}.style.top = '-75px';
NumberSpan{icon_class}.style.left = '-1px';
NumberSpan{icon_class}.style.backgroundColor = '';
NumberSpan{icon_class}.style.borderRadius = '5px';
NumberSpan{icon_class}.style.fontSize = '12pt';
NumberSpan{icon_class}.style.padding = '2px';
document.getElementsByClassName('{icon_class}')[0].appendChild(NumberSpan{icon_class});
</script>
"""
def set_number_icon(val):
if val!=0:
s = f"""
<script>
document.getElementsByClassName('{icon_class}')[0].childNodes[1].innerHTML = '{str(val)}';
document.getElementsByClassName('{icon_class}')[0].childNodes[1].style.backgroundColor = 'red';
</script>
"""
else:
s = f"""
<script>
console.log('ico1');
document.getElementsByClassName('{icon_class}')[0].childNodes[1].innerHTML = '';
document.getElementsByClassName('{icon_class}')[0].childNodes[1].style.backgroundColor = 'white';
console.log('ico2');
</script>
"""
html.object = s
pn.bind(set_number_icon, slider.param.value, watch=True)
pn.Row(svg,html,slider).servable()
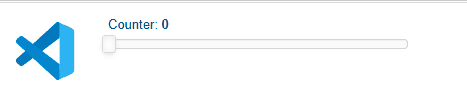